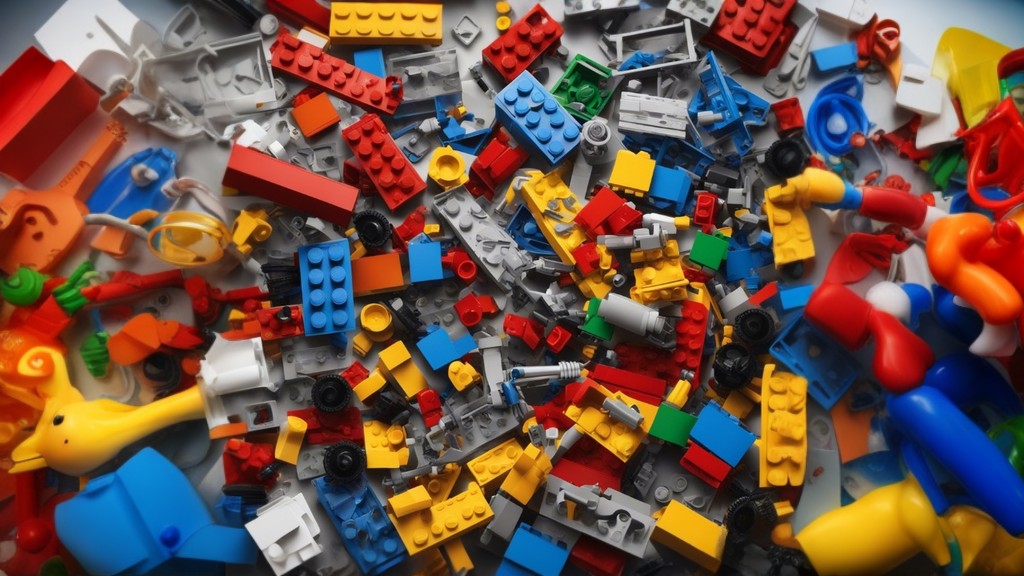
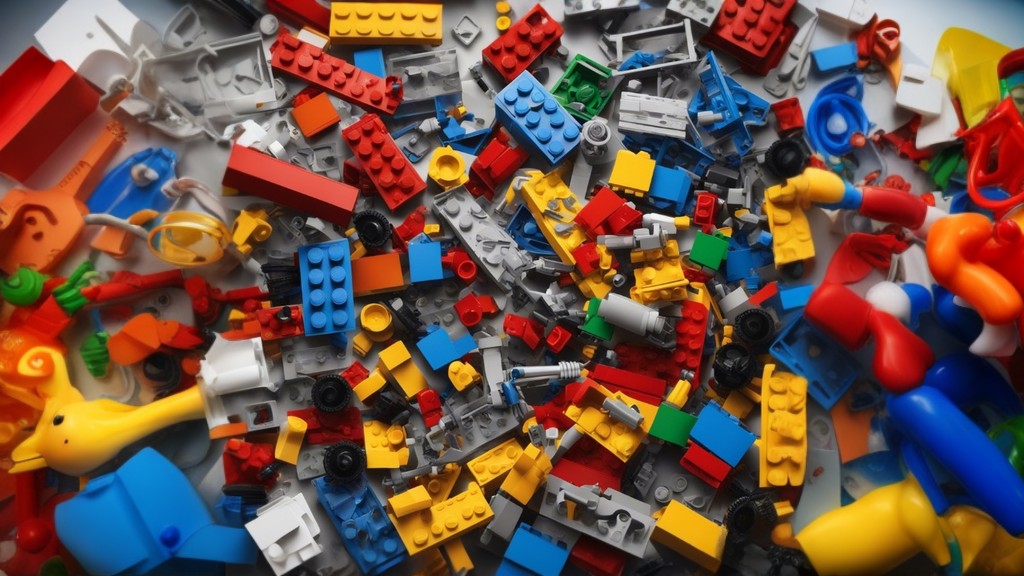
Table of content
TL;DR
Design patterns are essential tools for software developers. They provide proven solutions to common programming problems, making your code more efficient and maintainable. In this article, we’ll explore the world of design patterns, understand their importance, and learn how to implement them effectively.
Introduction
Design patterns are like blueprints for building software. They are tried-and-true solutions to recurring problems in software design. These patterns help developers create code that is not only robust but also easier to understand, modify, and maintain. Let’s dive deeper into the world of design patterns and discover why they are crucial in software development.
The Significance of Design Patterns
Code Reusability
Design patterns promote code reusability by providing templates for solving specific problems. Instead of reinventing the wheel each time you encounter a common issue, you can simply apply a design pattern that suits the situation. This saves time and effort in the long run.
Maintainability
Well-structured code is easier to maintain. Design patterns encourage a structured approach to development, making it simpler to debug and enhance your software. This is especially important when working on large projects with multiple team members.
Scalability
As your software grows, so do its complexities. Design patterns ensure that your code remains scalable. They allow you to add new features or modify existing ones without causing a ripple effect of bugs throughout your application.
Common Vocabulary
Design patterns provide a common vocabulary for developers. When everyone on your team understands these patterns, communication becomes more efficient. You can quickly convey your ideas and solutions using design pattern terminology.
Types of Design Patterns
There are several categories of design patterns, each serving a distinct purpose. Let’s take a look at some of the most commonly used ones:
Creational Patterns
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Examples include Singleton, Factory Method, and Builder patterns.
Singleton Design Pattern
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to that instance. In other words, it restricts the instantiation of a class to a single object and provides a way to access that instance from anywhere within the application.
1. Why Choose the Singleton Design Pattern:
There are several reasons to choose the Singleton design pattern:
Global Access: It allows a single, global point of access to an instance, which can be useful when you need to coordinate actions across the entire system.
Resource Management: Singletons are often used to manage resources like database connections or thread pools, ensuring that only one instance is responsible for managing these resources.
Configuration Settings: Singleton can be used to store and manage configuration settings for an application, making them easily accessible throughout the program.
Lazy Initialization: You can delay the creation of the Singleton instance until it is needed, which can be more efficient in terms of resource usage.
Controlled Access: It provides a way to control and restrict access to the instance, ensuring that multiple instances of the class cannot be created accidentally.
2. Real-World Examples:
Singleton pattern can be found in various real-world scenarios:
Database Connection Pooling: Database connection pooling frameworks often use the Singleton pattern to manage and reuse database connections efficiently across multiple parts of an application.
Logging: Logging systems that record events throughout an application can be implemented as a Singleton to ensure consistent and centralized logging.
Caching: Caching mechanisms, such as storing frequently used data in memory, are often implemented as Singleton objects to ensure that the cache is accessible and consistent throughout the application.
Print Spoolers: In operating systems, print spoolers are often implemented as Singleton objects to manage the printing process for all applications.
3. Code Example:
Here’s a simple example of implementing the Singleton pattern:
class Singleton {
static let shared = Singleton() // Static instance
private init() {} // Private constructor to prevent direct instantiation
func doSomething() {
print("Singleton is doing something.")
}
}
// Usage
let singletonInstance1 = Singleton.shared
let singletonInstance2 = Singleton.shared
singletonInstance1.doSomething() // Output: Singleton is doing something.
singletonInstance2.doSomething() // Output: Singleton is doing something.
// Both instances are the same
if singletonInstance1 === singletonInstance2 {
print("Both instances are the same.")
} else {
print("Instances are different.")
}
class Singleton {
static let shared = Singleton() // Static instance
private init() {} // Private constructor to prevent direct instantiation
func doSomething() {
print("Singleton is doing something.")
}
}
// Usage
let singletonInstance1 = Singleton.shared
let singletonInstance2 = Singleton.shared
singletonInstance1.doSomething() // Output: Singleton is doing something.
singletonInstance2.doSomething() // Output: Singleton is doing something.
// Both instances are the same
if singletonInstance1 === singletonInstance2 {
print("Both instances are the same.")
} else {
print("Instances are different.")
}
In this example,
Singleton
ensures that only one instance is created and provides global access through theshared
property. BothsingletonInstance1
andsingletonInstance2
point to the same instance, confirming that it’s a Singleton.
Factory Method Design Pattern
The Factory Method design pattern does provides an interface for creating objects but allows subclasses to alter the type of objects that will be created. It abstracts the process of object creation, promoting loose coupling and flexibility in your code.
1. Why Choose the Factory Method Design Pattern:
There are several reasons to choose the Factory Method design pattern:
Flexibility: It allows you to decouple the client code from the specific classes it needs to instantiate, making it easy to change or extend the types of objects that can be created without modifying the client code.
Encapsulation: The creation logic is encapsulated within the factory method, which helps keep the creation code clean and maintainable.
Subclassing: It’s particularly useful when you have a class hierarchy and want to delegate the responsibility of object creation to subclasses. Each subclass can create objects tailored to its specific requirements.
Testing: It simplifies unit testing, as you can substitute mock or stub factory methods to control object creation during testing.
2. Real-World Examples:
The Factory Method pattern can be found in various real-world scenarios:
Document Editors: Document editors like Microsoft Word use a Factory Method to create different types of documents (e.g., text documents, spreadsheets, presentations) based on user input.
GUI Libraries: Graphical user interface libraries often use the Factory Method to create platform-specific UI components (e.g., buttons, windows) without the client code needing to be aware of the underlying platform.
Game Development: In game development, the Factory Method can be used to create different types of game characters, weapons, or items based on the game’s design.
Plugin Systems: Applications with plugin systems may use the Factory Method to dynamically load and create plugin instances.
3. Code Example:
Here’s a simple example of implementing the Factory Method pattern:
// Product protocol
protocol Product {
func display()
}
// Concrete products
class ConcreteProductA: Product {
func display() {
print("Product A")
}
}
class ConcreteProductB: Product {
func display() {
print("Product B")
}
}
// Creator protocol
protocol Creator {
func createProduct() -> Product
}
// Concrete creators
class ConcreteCreatorA: Creator {
func createProduct() -> Product {
return ConcreteProductA()
}
}
class ConcreteCreatorB: Creator {
func createProduct() -> Product {
return ConcreteProductB()
}
}
// Usage
let creatorA: Creator = ConcreteCreatorA()
let creatorB: Creator = ConcreteCreatorB()
let productA = creatorA.createProduct()
let productB = creatorB.createProduct()
productA.display() // Output: Product A
productB.display() // Output: Product B
// Product protocol
protocol Product {
func display()
}
// Concrete products
class ConcreteProductA: Product {
func display() {
print("Product A")
}
}
class ConcreteProductB: Product {
func display() {
print("Product B")
}
}
// Creator protocol
protocol Creator {
func createProduct() -> Product
}
// Concrete creators
class ConcreteCreatorA: Creator {
func createProduct() -> Product {
return ConcreteProductA()
}
}
class ConcreteCreatorB: Creator {
func createProduct() -> Product {
return ConcreteProductB()
}
}
// Usage
let creatorA: Creator = ConcreteCreatorA()
let creatorB: Creator = ConcreteCreatorB()
let productA = creatorA.createProduct()
let productB = creatorB.createProduct()
productA.display() // Output: Product A
productB.display() // Output: Product B
In this example, we have a
Product
protocol representing the products to be created. Concrete productsConcreteProductA
andConcreteProductB
implement this protocol. TheCreator
protocol defines the factory methodcreateProduct()
, which concrete creators (ConcreteCreatorA
andConcreteCreatorB
) implement to create specific product instances. This demonstrates how the Factory Method pattern allows you to create different types of objects through a common interface.
Builder Design Pattern
The Builder design pattern is a creational pattern that separates the construction of a complex object from its representation. It allows you to create an object step by step, providing fine-grained control over its construction process. The Builder pattern is especially useful when an object has a large number of possible configurations, and you want to simplify the creation process.
1. Why Choose the Builder Design Pattern:
Here are some reasons to choose the Builder design pattern:
Complex Object Creation: When you need to create objects with a complex set of parameters, especially when there are optional or variable parts, the Builder pattern can simplify the process and improve code readability.
Parameter Flexibility: Builders allow you to set parameters in a step-by-step manner, making it easy to configure objects with different combinations of parameters.
Immutability: The Builder pattern is often used in combination with immutability, ensuring that once an object is constructed, it cannot be modified, which can be important for thread safety.
Readability: It can improve code readability, as the construction steps are clearly defined, and the client code is more self-explanatory.
2. Real-World Examples:
The Builder pattern can be found in various real-world scenarios:
HTML Document Generation: Building an HTML document can involve creating various elements (head, body, paragraphs, links, etc.), and their attributes. A builder can help construct complex HTML structures step by step.
Database Query Builders: Libraries or frameworks for database access often use the Builder pattern to construct SQL queries or other database operations with various conditions, joins, and filters.
Meal Ordering in Restaurants: In a restaurant, a meal can be customized with different components (appetizers, main courses, sides, drinks, etc.). A builder can be used to create a custom meal with specific choices.
Configuration Builders: When configuring software components, such as network clients or application settings, builders can provide a convenient way to set various options and parameters.
3. Code Example:
Here’s a simple example of implementing the Builder pattern for constructing a custom burger in a fast-food restaurant:
// Product
struct Burger {
var patty: String
var cheese: Bool
var lettuce: Bool
var tomato: Bool
var pickles: Bool
}
// Builder
protocol BurgerBuilder {
func addPatty(_ patty: String)
func addCheese()
func addLettuce()
func addTomato()
func addPickles()
func build() -> Burger
}
// Concrete Builder
class CustomBurgerBuilder: BurgerBuilder {
private var burger = Burger(patty: "Beef", cheese: false, lettuce: false, tomato: false, pickles: false)
func addPatty(_ patty: String) {
burger.patty = patty
}
func addCheese() {
burger.cheese = true
}
func addLettuce() {
burger.lettuce = true
}
func addTomato() {
burger.tomato = true
}
func addPickles() {
burger.pickles = true
}
func build() -> Burger {
return burger
}
}
// Director
class BurgerChef {
private var builder: BurgerBuilder
init(builder: BurgerBuilder) {
self.builder = builder
}
func constructBurger() -> Burger {
builder.addPatty("Chicken")
builder.addCheese()
builder.addLettuce()
builder.addTomato()
builder.addPickles()
return builder.build()
}
}
// Usage
let customBurgerBuilder = CustomBurgerBuilder()
let chef = BurgerChef(builder: customBurgerBuilder)
let customBurger = chef.constructBurger()
print("Custom Burger:")
print("Patty: (customBurger.patty)")
print("Cheese: (customBurger.cheese)")
print("Lettuce: (customBurger.lettuce)")
print("Tomato: (customBurger.tomato)")
print("Pickles: (customBurger.pickles)")
// Product
struct Burger {
var patty: String
var cheese: Bool
var lettuce: Bool
var tomato: Bool
var pickles: Bool
}
// Builder
protocol BurgerBuilder {
func addPatty(_ patty: String)
func addCheese()
func addLettuce()
func addTomato()
func addPickles()
func build() -> Burger
}
// Concrete Builder
class CustomBurgerBuilder: BurgerBuilder {
private var burger = Burger(patty: "Beef", cheese: false, lettuce: false, tomato: false, pickles: false)
func addPatty(_ patty: String) {
burger.patty = patty
}
func addCheese() {
burger.cheese = true
}
func addLettuce() {
burger.lettuce = true
}
func addTomato() {
burger.tomato = true
}
func addPickles() {
burger.pickles = true
}
func build() -> Burger {
return burger
}
}
// Director
class BurgerChef {
private var builder: BurgerBuilder
init(builder: BurgerBuilder) {
self.builder = builder
}
func constructBurger() -> Burger {
builder.addPatty("Chicken")
builder.addCheese()
builder.addLettuce()
builder.addTomato()
builder.addPickles()
return builder.build()
}
}
// Usage
let customBurgerBuilder = CustomBurgerBuilder()
let chef = BurgerChef(builder: customBurgerBuilder)
let customBurger = chef.constructBurger()
print("Custom Burger:")
print("Patty: (customBurger.patty)")
print("Cheese: (customBurger.cheese)")
print("Lettuce: (customBurger.lettuce)")
print("Tomato: (customBurger.tomato)")
print("Pickles: (customBurger.pickles)")
In this example, the
BurgerBuilder
protocol defines the construction steps for building a custom burger. TheCustomBurgerBuilder
class is a concrete builder that implements these steps. TheBurgerChef
class acts as a director, orchestrating the construction process. This demonstrates how the Builder pattern can be used to create complex objects with varying configurations.
Structural Patterns
Structural patterns focus on how objects are composed to form larger structures. Some well-known structural patterns are Adapter, Decorator, and Facade patterns.
Adapter Design Pattern
The Adapter design pattern is a structural pattern that allows objects with incompatible interfaces to work together. It acts as a bridge between two interfaces, converting one interface into another, making them compatible without modifying their source code. The Adapter pattern is particularly useful when integrating existing systems, classes, or components that have differing interfaces.
1. Why Choose the Adapter Design Pattern:
There are several reasons to choose the Adapter design pattern:
Legacy System Integration: When you need to integrate or reuse older components or systems with different interfaces in a new application, the Adapter pattern allows you to do so without changing the existing code.
Third-Party Library Compatibility: When you want to use a third-party library or API that doesn’t conform to your application’s interface, you can create an adapter to make it compatible.
Interface Standardization: It helps in standardizing interfaces across various components or classes in your system, making it easier to maintain and understand.
Code Reuse: Adapters allow you to reuse existing code or components that would otherwise be unusable due to interface mismatches.
2. Real-World Examples:
The Adapter pattern can be found in various real-world software scenarios:
USB Adapters: USB adapters are a common example. They allow you to connect USB devices with different connectors (e.g., USB-A to USB-C) to your computer.
Audio/Video Adapters: Adapters are used to connect audio and video devices with different connectors or formats, such as HDMI to VGA adapters.
Database Adapters: Database adapters are often used to convert between different database interfaces and allow an application to work with various database management systems.
Web Service Integration: When integrating with external web services or APIs, you may need to create adapters to transform the service’s response into a format that your application can work with.
3. Code Example:
Here’s a simple example of implementing the Adapter pattern for adapting Celsius to Fahrenheit temperature conversion:
// Adaptee: This is the class with the incompatible interface
class CelsiusTemperature {
func getCelsiusTemperature() -> Double {
return 25.0
}
}
// Target: This is the interface we want to work with (in Fahrenheit)
protocol Temperature {
func getTemperature() -> Double
}
// Adapter: This adapts Celsius to the Temperature interface
class CelsiusToFahrenheitAdapter: Temperature {
private let celsiusTemperature: CelsiusTemperature
init(celsiusTemperature: CelsiusTemperature) {
self.celsiusTemperature = celsiusTemperature
}
func getTemperature() -> Double {
let celsius = celsiusTemperature.getCelsiusTemperature()
let fahrenheit = (celsius * 9.0/5.0) + 32.0
return fahrenheit
}
}
// Client code
let celsiusTemperature = CelsiusTemperature()
let temperatureAdapter = CelsiusToFahrenheitAdapter(celsiusTemperature: celsiusTemperature)
print("Temperature in Fahrenheit: (temperatureAdapter.getTemperature())")
// Adaptee: This is the class with the incompatible interface
class CelsiusTemperature {
func getCelsiusTemperature() -> Double {
return 25.0
}
}
// Target: This is the interface we want to work with (in Fahrenheit)
protocol Temperature {
func getTemperature() -> Double
}
// Adapter: This adapts Celsius to the Temperature interface
class CelsiusToFahrenheitAdapter: Temperature {
private let celsiusTemperature: CelsiusTemperature
init(celsiusTemperature: CelsiusTemperature) {
self.celsiusTemperature = celsiusTemperature
}
func getTemperature() -> Double {
let celsius = celsiusTemperature.getCelsiusTemperature()
let fahrenheit = (celsius * 9.0/5.0) + 32.0
return fahrenheit
}
}
// Client code
let celsiusTemperature = CelsiusTemperature()
let temperatureAdapter = CelsiusToFahrenheitAdapter(celsiusTemperature: celsiusTemperature)
print("Temperature in Fahrenheit: (temperatureAdapter.getTemperature())")
In this example,
CelsiusTemperature
is the Adaptee class with an incompatible interface, representing temperature in Celsius. TheTemperature
protocol is the target interface we want to work with, representing temperature in Fahrenheit. TheCelsiusToFahrenheitAdapter
class acts as the adapter, converting Celsius to Fahrenheit. The client code can work with theTemperature
interface, and the adapter bridges the gap between the incompatible interfaces.
Composite Design Pattern
The Composite design pattern is a structural pattern that lets you compose objects into tree structures to represent part-whole hierarchies. It allows clients to treat individual objects and compositions of objects (composites) uniformly. This pattern is particularly useful when you want to represent objects and their compositions hierarchically, enabling you to work with both individual objects and complex structures of objects in a consistent manner.
1. Why Choose the Composite Design Pattern:
There are several reasons to choose the Composite design pattern:
Hierarchy Representation: When you need to represent complex hierarchies or tree structures of objects, the Composite pattern provides a consistent way to do so, allowing you to treat individual objects and compositions uniformly.
Simplifying Client Code: Clients can interact with both leaf objects (individual objects) and composite objects (composed of multiple objects) using a single interface. This simplifies client code by eliminating the need to distinguish between individual and composite elements.
Recursive Behavior: The pattern enables recursive behavior, allowing you to apply operations to the entire hierarchy or its individual components.
Scalability: It makes it easy to add new components to the hierarchy, as both leaf and composite objects adhere to the same interface.
2. Real-World Examples:
The Composite pattern can be found in various real-world scenarios:
Graphic Design Software: In graphic design software, objects like shapes, lines, and groups of shapes can be organized hierarchically using the Composite pattern. This allows users to manipulate individual shapes or entire compositions.
File Systems: File systems are often organized hierarchically, with directories containing files and subdirectories. The Composite pattern can be used to represent and manipulate file systems in a consistent way.
GUI Libraries: Graphical user interface libraries use the Composite pattern to represent complex GUI components like windows, panels, buttons, and containers, allowing users to create and manipulate user interfaces.
Organization Charts: Organizational structures, such as company hierarchies, can be represented using the Composite pattern. Employees, departments, and divisions can all be treated uniformly.
3. Code Example:
Here’s a simple example of implementing the Composite pattern to represent and manipulate a hierarchy of geometric shapes:
// Component interface
protocol Shape {
func draw()
}
// Leaf class
class Circle: Shape {
func draw() {
print("Drawing a circle")
}
}
class Square: Shape {
func draw() {
print("Drawing a square")
}
}
// Composite class
class Group: Shape {
private var shapes: [Shape] = []
func add(shape: Shape) {
shapes.append(shape)
}
func draw() {
print("Drawing a group of shapes:")
for shape in shapes {
shape.draw()
}
}
}
// Client code
let circle1 = Circle()
let circle2 = Circle()
let square = Square()
let group = Group()
group.add(shape: circle1)
group.add(shape: circle2)
group.add(shape: square)
group.draw()
// Component interface
protocol Shape {
func draw()
}
// Leaf class
class Circle: Shape {
func draw() {
print("Drawing a circle")
}
}
class Square: Shape {
func draw() {
print("Drawing a square")
}
}
// Composite class
class Group: Shape {
private var shapes: [Shape] = []
func add(shape: Shape) {
shapes.append(shape)
}
func draw() {
print("Drawing a group of shapes:")
for shape in shapes {
shape.draw()
}
}
}
// Client code
let circle1 = Circle()
let circle2 = Circle()
let square = Square()
let group = Group()
group.add(shape: circle1)
group.add(shape: circle2)
group.add(shape: square)
group.draw()
In this example,
Shape
is the component interface that both leaf objects (Circle
andSquare
) and composite objects (Group
) implement.Group
can contain a collection of shapes, including other groups. This structure allows you to draw individual shapes or entire compositions in a consistent manner, demonstrating the Composite pattern’s ability to work with hierarchical structures of objects.
Behavioral Patterns
Behavioral patterns define the ways in which objects interact and communicate. The Observer, Strategy, and Command patterns fall into this category.
Chain of Responsibility Design Pattern
The Chain of Responsibility design pattern is a behavioral pattern that allows you to pass requests along a chain of handlers. Each handler decides either to process the request or pass it to the next handler in the chain. This pattern decouples the sender of a request from its receivers, enabling multiple objects to handle the request without the sender needing to know which object will eventually process it. It promotes flexibility, scalability, and the avoidance of hard-coded dependencies between objects.
1. Why Choose the Chain of Responsibility Design Pattern:
There are several reasons to choose the Chain of Responsibility design pattern:
Loose Coupling: It promotes loose coupling between the sender of a request and its potential receivers, making the system more flexible and easier to extend or modify.
Responsibility Assignment: It allows you to define a set of handlers, each responsible for specific types of requests, making the codebase more organized and modular.
Dynamic Handling: Handlers can be added or removed dynamically at runtime, enabling you to change the order or composition of the chain without modifying the client code.
Single Responsibility Principle (SRP): It encourages adhering to the SRP by ensuring that each handler class has a single, well-defined responsibility.
2. Real-World Examples:
The Chain of Responsibility pattern can be found in various real-world scenarios:
Logging Systems: In logging frameworks, loggers can be organized in a chain, with each logger deciding whether to handle a log message or pass it to the next logger in the chain based on log levels or other criteria.
Middleware in Web Frameworks: In web frameworks like Express.js (Node.js) or Django (Python), middleware functions form a chain to process HTTP requests. Each middleware component can choose to handle the request or pass it to the next middleware.
Event Handling in GUI Libraries: GUI libraries use event handling mechanisms where event handlers are organized in a chain. The event system routes events through this chain to handle user input, such as mouse clicks or keyboard presses.
Authorization and Authentication: In authentication and authorization systems, multiple checks may be performed by different handlers to grant or deny access to certain resources.
3. Code Example:
Here’s a simple example of implementing the Chain of Responsibility pattern to process purchase requests:
// Request class
class PurchaseRequest {
var amount: Double
init(amount: Double) {
self.amount = amount
}
}
// Handler protocol
protocol PurchaseHandler {
var successor: PurchaseHandler? { get set }
func processRequest(request: PurchaseRequest)
}
// Concrete Handlers
class Manager: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 1000 {
print("Manager approved the purchase request of $(request.amount)")
} else if let successor = successor {
successor.processRequest(request: request)
} else {
print("Request cannot be approved.")
}
}
}
class Director: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 5000 {
print("Director approved the purchase request of $(request.amount)")
} else if let successor = successor {
successor.processRequest(request: request)
} else {
print("Request cannot be approved.")
}
}
}
class CEO: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 10000 {
print("CEO approved the purchase request of $(request.amount)")
} else {
print("Request cannot be approved.")
}
}
}
// Usage
let manager = Manager()
let director = Director()
let ceo = CEO()
manager.successor = director
director.successor = ceo
let request1 = PurchaseRequest(amount: 800)
let request2 = PurchaseRequest(amount: 4500)
let request3 = PurchaseRequest(amount: 12000)
manager.processRequest(request: request1)
manager.processRequest(request: request2)
manager.processRequest(request: request3)
// Request class
class PurchaseRequest {
var amount: Double
init(amount: Double) {
self.amount = amount
}
}
// Handler protocol
protocol PurchaseHandler {
var successor: PurchaseHandler? { get set }
func processRequest(request: PurchaseRequest)
}
// Concrete Handlers
class Manager: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 1000 {
print("Manager approved the purchase request of $(request.amount)")
} else if let successor = successor {
successor.processRequest(request: request)
} else {
print("Request cannot be approved.")
}
}
}
class Director: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 5000 {
print("Director approved the purchase request of $(request.amount)")
} else if let successor = successor {
successor.processRequest(request: request)
} else {
print("Request cannot be approved.")
}
}
}
class CEO: PurchaseHandler {
var successor: PurchaseHandler?
func processRequest(request: PurchaseRequest) {
if request.amount <= 10000 {
print("CEO approved the purchase request of $(request.amount)")
} else {
print("Request cannot be approved.")
}
}
}
// Usage
let manager = Manager()
let director = Director()
let ceo = CEO()
manager.successor = director
director.successor = ceo
let request1 = PurchaseRequest(amount: 800)
let request2 = PurchaseRequest(amount: 4500)
let request3 = PurchaseRequest(amount: 12000)
manager.processRequest(request: request1)
manager.processRequest(request: request2)
manager.processRequest(request: request3)
In this example,
PurchaseHandler
defines the handler protocol, and concrete handlers (Manager
,Director
, andCEO
) implement this protocol. Requests for purchase are processed through the chain of handlers, and each handler decides whether to approve or pass the request to the next handler in the chain based on the request amount.
Interpreter Design Pattern
The Interpreter design pattern is a behavioral pattern that is used to define a grammar for a language and provides an interpreter to interpret sentences in that language. It allows you to build a language processor or parser for a specific domain-specific language (DSL) or notation. The Interpreter pattern is particularly useful when you have a language to represent and need to evaluate expressions or statements written in that language.
1. Why Choose the Interpreter Design Pattern:
Here are some reasons to choose the Interpreter design pattern:
Domain-Specific Languages: When you need to define and work with domain-specific languages tailored for specific tasks, the Interpreter pattern can help you parse and evaluate expressions or statements in those languages.
Separation of Concerns: It separates the parsing and evaluation concerns, making it easier to maintain and extend the language interpreter independently.
Extensibility: New expressions or statements can be added to the language interpreter without modifying the existing codebase, promoting extensibility.
Readability: For complex parsing tasks or when dealing with complex syntax, an interpreter can improve code readability by abstracting away the parsing details.
2. Real-World Examples:
The Interpreter pattern can be found in various real-world scenarios:
Regular Expressions: Many programming languages use regular expressions, which can be thought of as a simple domain-specific language for pattern matching. An interpreter is used to evaluate and match strings against regular expressions.
Database Query Languages: SQL (Structured Query Language) interpreters parse and execute SQL queries. Each SQL statement represents a sentence in the SQL language, and the interpreter evaluates them accordingly.
Mathematical Expression Evaluation: Scientific and engineering software often uses interpreters to evaluate mathematical expressions written in various notations (e.g., postfix, infix, prefix).
Configuration Languages: Some software uses interpreters to read and interpret configuration files that specify settings or behaviors using a custom DSL.
3. Code Example:
Here’s a simple example of implementing the Interpreter pattern to evaluate mathematical expressions in postfix notation:
// Context
struct Context {
var variables: [String: Double] = [:]
}
// Abstract Expression
protocol Expression {
func interpret(context: Context) -> Double
}
// Terminal Expressions
struct Number: Expression {
private let value: Double
init(_ value: Double) {
self.value = value
}
func interpret(context: Context) -> Double {
return value
}
}
struct Variable: Expression {
private let variableName: String
init(_ variableName: String) {
self.variableName = variableName
}
func interpret(context: Context) -> Double {
return context.variables[variableName] ?? 0.0
}
}
// Non-terminal Expressions
struct Addition: Expression {
private let left: Expression
private let right: Expression
init(_ left: Expression, _ right: Expression) {
self.left = left
self.right = right
}
func interpret(context: Context) -> Double {
return left.interpret(context: context) + right.interpret(context: context)
}
}
struct Subtraction: Expression {
private let left: Expression
private let right: Expression
init(_ left: Expression, _ right: Expression) {
self.left = left
self.right = right
}
func interpret(context: Context) -> Double {
return left.interpret(context: context) - right.interpret(context: context)
}
}
// Usage
let context = Context()
context.variables["x"] = 5.0
context.variables["y"] = 7.0
let expression = Addition(Variable("x"), Subtraction(Number(10), Variable("y")))
let result = expression.interpret(context: context)
print("Result: (result)") // Output: Result: -3.0
// Context
struct Context {
var variables: [String: Double] = [:]
}
// Abstract Expression
protocol Expression {
func interpret(context: Context) -> Double
}
// Terminal Expressions
struct Number: Expression {
private let value: Double
init(_ value: Double) {
self.value = value
}
func interpret(context: Context) -> Double {
return value
}
}
struct Variable: Expression {
private let variableName: String
init(_ variableName: String) {
self.variableName = variableName
}
func interpret(context: Context) -> Double {
return context.variables[variableName] ?? 0.0
}
}
// Non-terminal Expressions
struct Addition: Expression {
private let left: Expression
private let right: Expression
init(_ left: Expression, _ right: Expression) {
self.left = left
self.right = right
}
func interpret(context: Context) -> Double {
return left.interpret(context: context) + right.interpret(context: context)
}
}
struct Subtraction: Expression {
private let left: Expression
private let right: Expression
init(_ left: Expression, _ right: Expression) {
self.left = left
self.right = right
}
func interpret(context: Context) -> Double {
return left.interpret(context: context) - right.interpret(context: context)
}
}
// Usage
let context = Context()
context.variables["x"] = 5.0
context.variables["y"] = 7.0
let expression = Addition(Variable("x"), Subtraction(Number(10), Variable("y")))
let result = expression.interpret(context: context)
print("Result: (result)") // Output: Result: -3.0
In this example, the Interpreter pattern is used to evaluate mathematical expressions in postfix notation.
Expression
represents the abstract syntax tree (AST), and various concrete terminal and non-terminal expressions are implemented. TheContext
stores variable values, and expressions are interpreted within this context.
Observer Design Pattern
The Observer design pattern is a behavioral pattern that defines a one-to-many dependency between objects. In this pattern, when one object (the subject or publisher) changes its state, all dependent objects (observers or subscribers) are notified and updated automatically. The Observer pattern is commonly used for implementing distributed event handling systems, where an object needs to notify multiple other objects of its state changes without making assumptions about who or what those objects are.
1. Why Choose the Observer Design Pattern:
There are several reasons to choose the Observer design pattern:
Decoupling: It promotes loose coupling between the subject and its observers, allowing them to interact without having detailed knowledge of each other.
Flexibility: The Observer pattern allows you to add or remove observers dynamically. New observers can be added without modifying existing code, making it highly extensible.
Event Handling: It’s an essential pattern for implementing event-driven systems, where one object’s state changes trigger actions in multiple other objects.
Maintainability: By separating concerns and reducing dependencies, it makes the codebase more maintainable and easier to understand.
2. Real-World Examples:
The Observer pattern can be found in various real-world scenarios:
GUI Frameworks: Graphical user interface frameworks use the Observer pattern extensively. For example, when you click a button in a GUI, multiple UI components (e.g., text fields, labels, and buttons) need to respond to the event, and the Observer pattern facilitates this.
Stock Market Updates: In financial systems, stock market updates are broadcasted to multiple subscribers (e.g., traders, analysts) in real-time. The Observer pattern ensures that all interested parties receive updates when stock prices change.
Publish-Subscribe Messaging: Message brokers and pub-sub systems rely on the Observer pattern to distribute messages to multiple subscribers based on topics or channels.
Weather Monitoring Systems: Weather stations collect data and broadcast it to multiple display devices, such as digital weather displays, mobile apps, or websites, using the Observer pattern.
3. Code Example:
Here’s a simple example of implementing the Observer pattern to create a weather monitoring system:
// Observer protocol
protocol Observer {
func update(temperature: Double, humidity: Double)
}
// Subject (Observable)
class WeatherStation {
private var temperature: Double = 0.0
private var humidity: Double = 0.0
private var observers: [Observer] = []
func setMeasurements(temperature: Double, humidity: Double) {
self.temperature = temperature
self.humidity = humidity
notifyObservers()
}
func addObserver(observer: Observer) {
observers.append(observer)
}
func removeObserver(observer: Observer) {
if let index = observers.firstIndex(where: { $0 === observer }) {
observers.remove(at: index)
}
}
private func notifyObservers() {
for observer in observers {
observer.update(temperature: temperature, humidity: humidity)
}
}
}
// Concrete Observers
class Display: Observer {
func update(temperature: Double, humidity: Double) {
print("Display: Temperature (temperature)°C, Humidity (humidity)%")
}
}
class Logger: Observer {
func update(temperature: Double, humidity: Double) {
print("Logger: Recording data - Temperature (temperature)°C, Humidity (humidity)%")
}
}
// Usage
let weatherStation = WeatherStation()
let display = Display()
let logger = Logger()
weatherStation.addObserver(observer: display)
weatherStation.addObserver(observer: logger)
weatherStation.setMeasurements(temperature: 25.5, humidity: 60)
weatherStation.setMeasurements(temperature: 28.0, humidity: 55)
weatherStation.removeObserver(observer: display)
weatherStation.setMeasurements(temperature: 30.0, humidity: 50)
// Observer protocol
protocol Observer {
func update(temperature: Double, humidity: Double)
}
// Subject (Observable)
class WeatherStation {
private var temperature: Double = 0.0
private var humidity: Double = 0.0
private var observers: [Observer] = []
func setMeasurements(temperature: Double, humidity: Double) {
self.temperature = temperature
self.humidity = humidity
notifyObservers()
}
func addObserver(observer: Observer) {
observers.append(observer)
}
func removeObserver(observer: Observer) {
if let index = observers.firstIndex(where: { $0 === observer }) {
observers.remove(at: index)
}
}
private func notifyObservers() {
for observer in observers {
observer.update(temperature: temperature, humidity: humidity)
}
}
}
// Concrete Observers
class Display: Observer {
func update(temperature: Double, humidity: Double) {
print("Display: Temperature (temperature)°C, Humidity (humidity)%")
}
}
class Logger: Observer {
func update(temperature: Double, humidity: Double) {
print("Logger: Recording data - Temperature (temperature)°C, Humidity (humidity)%")
}
}
// Usage
let weatherStation = WeatherStation()
let display = Display()
let logger = Logger()
weatherStation.addObserver(observer: display)
weatherStation.addObserver(observer: logger)
weatherStation.setMeasurements(temperature: 25.5, humidity: 60)
weatherStation.setMeasurements(temperature: 28.0, humidity: 55)
weatherStation.removeObserver(observer: display)
weatherStation.setMeasurements(temperature: 30.0, humidity: 50)
In this example, the
WeatherStation
class represents the subject, while theDisplay
andLogger
classes are concrete observers. TheWeatherStation
notifies its observers whenever the weather measurements change. Observers likeDisplay
andLogger
respond to these updates by implementing theupdate
method.
Implementing Design Patterns
Choose the Right Pattern
Selecting the appropriate design pattern for your problem is crucial. Take time to understand the problem thoroughly and then choose the pattern that best fits the situation.
Study and Practice
Before implementing a design pattern, study it thoroughly. Understand its structure, benefits, and potential drawbacks. Practice by applying the pattern to smaller projects to gain confidence.
Documentation
Documentation is key when using design patterns. Ensure that your team is well-informed about the pattern you are implementing. Document the pattern’s usage and any deviations from the standard implementation.
Refactoring
Don’t be afraid to refactor your code when necessary. Design patterns may evolve as your project progresses, so be open to making improvements based on new requirements or insights.
Conclusion
In the world of software development, design patterns are your secret weapons for building robust, maintainable, and scalable applications. By incorporating these patterns into your coding repertoire, you’ll streamline your development process and enhance your team’s productivity. So, start mastering design patterns today and witness the positive impact on your software projects.